mirror of
https://github.com/AUTOMATIC1111/stable-diffusion-webui.git
synced 2025-02-14 01:22:58 +08:00
Updated API (markdown)
parent
6a68e7b965
commit
f690be5a19
32
API.md
32
API.md
@ -4,11 +4,9 @@
|
||||
- example in your "webui-user.bat": `set COMMANDLINE_ARGS=--api`
|
||||
- This enables the api which can be reviewed at http://127.0.0.1:7860/docs (or whever the URL is + /docs)
|
||||
The basic ones I'm interested in are these two. Let's just focus only on ` /sdapi/v1/txt2img`
|
||||
|
||||
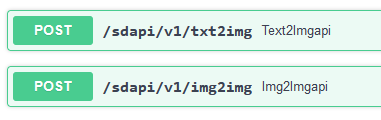
|
||||
|
||||
- When you expand that tab, it gives an example of a payload to send to the API. I used this often as reference.
|
||||
|
||||
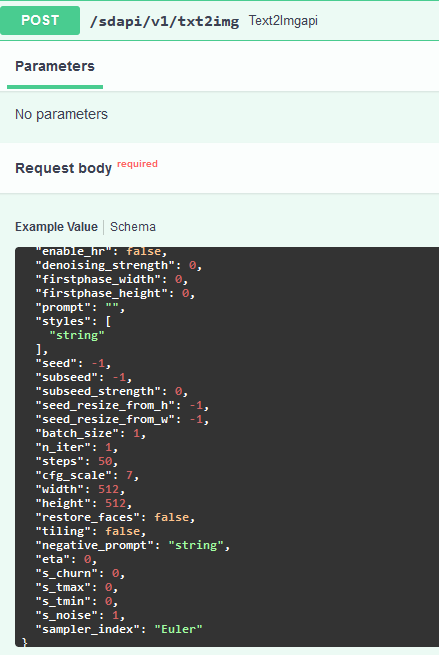
|
||||
|
||||
------
|
||||
@ -36,14 +34,17 @@ If we execute this code, the web ui will generate an image based on the payload.
|
||||
- "images" is the generated image, which is what I want mostly. There's no link or anything; it's a giant string of random characters, apparently we have to decode it. This is how I do it:
|
||||
```
|
||||
for i in r['images']:
|
||||
image = Image.open(io.BytesIO(base64.b64decode(i.split(",",1)[1])))
|
||||
image = Image.open(io.BytesIO(base64.b64decode(i.split(",",1)[0])))
|
||||
```
|
||||
- With that, we have an image in the `image` variable that we can work with, for example saving it with `image.save('output.png')`.
|
||||
- "parameters" shows what was sent to the API, which could be useful, but what I want in this case is "info". I use it to insert metadata into the image, so I can drop it into web ui PNG Info. For that, I'll backtrack a little bit and pull what I need, which is nested in "info"
|
||||
- "parameters" shows what was sent to the API, which could be useful, but what I want in this case is "info". I use it to insert metadata into the image, so I can drop it into web ui PNG Info. For that, I can access the `/sdapi/v1/png-info` API. I'll need to feed the image I got above into it.
|
||||
```
|
||||
load_r = json.loads(r['info'])
|
||||
meta = load_r["infotexts"][0]
|
||||
png_payload = {
|
||||
"image": "data:image/png;base64," + i
|
||||
}
|
||||
response2 = requests.post(url=f'http://127.0.0.1:7860/sdapi/v1/png-info', json=png_payload)
|
||||
```
|
||||
After that, I can get the information with `response2.json().get("info")`
|
||||
|
||||
------
|
||||
|
||||
@ -65,28 +66,31 @@ payload = {
|
||||
response = requests.post(url=f'{url}/sdapi/v1/txt2img', json=payload)
|
||||
|
||||
r = response.json()
|
||||
load_r = json.loads(r['info'])
|
||||
meta = load_r["infotexts"][0]
|
||||
|
||||
for i in r['images']:
|
||||
image = Image.open(io.BytesIO(base64.b64decode(i.split(",",1)[1])))
|
||||
image = Image.open(io.BytesIO(base64.b64decode(i.split(",",1)[0])))
|
||||
|
||||
png_payload = {
|
||||
"image": "data:image/png;base64," + i
|
||||
}
|
||||
response2 = requests.post(url=f'{url}/sdapi/v1/png-info', json=png_payload)
|
||||
|
||||
pnginfo = PngImagePlugin.PngInfo()
|
||||
pnginfo.add_text("parameters", meta)
|
||||
pnginfo.add_text("parameters", response2.json().get("info"))
|
||||
image.save('output.png', pnginfo=pnginfo)
|
||||
```
|
||||
- Import the things I need
|
||||
- define the url and the payload to send
|
||||
- send said payload to said url through the API
|
||||
- when I get the response, grab png info from "infotexts" inside of "info"
|
||||
- then grab "images" and decode it
|
||||
- in a loop grab "images" and decode it
|
||||
- for each image, send it to png info API and get that info back
|
||||
- define a plugin to add png info, then add the png info I defined into it
|
||||
- at the end here, save the image with the png info
|
||||
|
||||
This is as of commit [e725474](https://github.com/AUTOMATIC1111/stable-diffusion-webui/commit/e7254746bbfbff45099db44a8d4d25dd6181877d)
|
||||
This is as of commit [ac08562](https://github.com/AUTOMATIC1111/stable-diffusion-webui/commit/ac085628540d0ec6a988fad93f5b8f2154209571)
|
||||
|
||||
For a more complete implementation of a frontend, my Discord bot is [here](https://github.com/Kilvoctu/aiyabot) if anyone wants to look at it as an example. Most of the action happens in stablecog.py. There are many comments explaining what each code does.
|
||||
|
||||
|
||||
------
|
||||
|
||||
This guide can be found in [discussions](https://github.com/AUTOMATIC1111/stable-diffusion-webui/discussions/3734) page.
|
Loading…
Reference in New Issue
Block a user